« 1.4: Variables |
1.6: Disk Space Analyser Task »
Arithmetic Operators
Variables are far more useful when you perform arithmetic operations using them. Add the following code to your “variables” sketch:
...
rect(x,y, w,h)
print(x + 2)
I’m guessing that did exactly what you expected? You can also subtract:
...
rect(x,y, w,h)
print(x + 2) # displays 3
print(x - 2) # displays -1
Multiplication is performed using the *
operator:
...
print(x * 5) # displays 5
Now add the code below, but before hitting run, see if you can predict what the total is:
...
print(1 + 2 * 3) # displays ???
The console displays a 7
– and not a 9
– because multiplication occurs before addition. Certain operators take precedence over others. Remember BEDMAS? Or BODMAS (depending on where you’re from)? It’s an acronym to help you recall the order of operations. If you want to override this order, use brackets:
...
print(1 + 2 * 3) # displays 7
print((1 + 2) * 3) # displays 9
For division, use a forward-slash:
...
print(4 / 2) # displays 2
Be aware, though, that dividing two integers always produces an integer result (integers are ‘whole’ numbers, as opposed to those with a decimal point). For example:
...
print(3 / 2) # displays 1
Python discards any decimal digits, effectively ‘rounding-down’ the result. To allow decimal results, define at least one of your operands using a decimal point:
...
print(3 / 2.0) # displays 1.5
Decimal numbers are usually referred to as floating point, or float values, in programming terminology.
Of course, division by zero operations will result in errors:
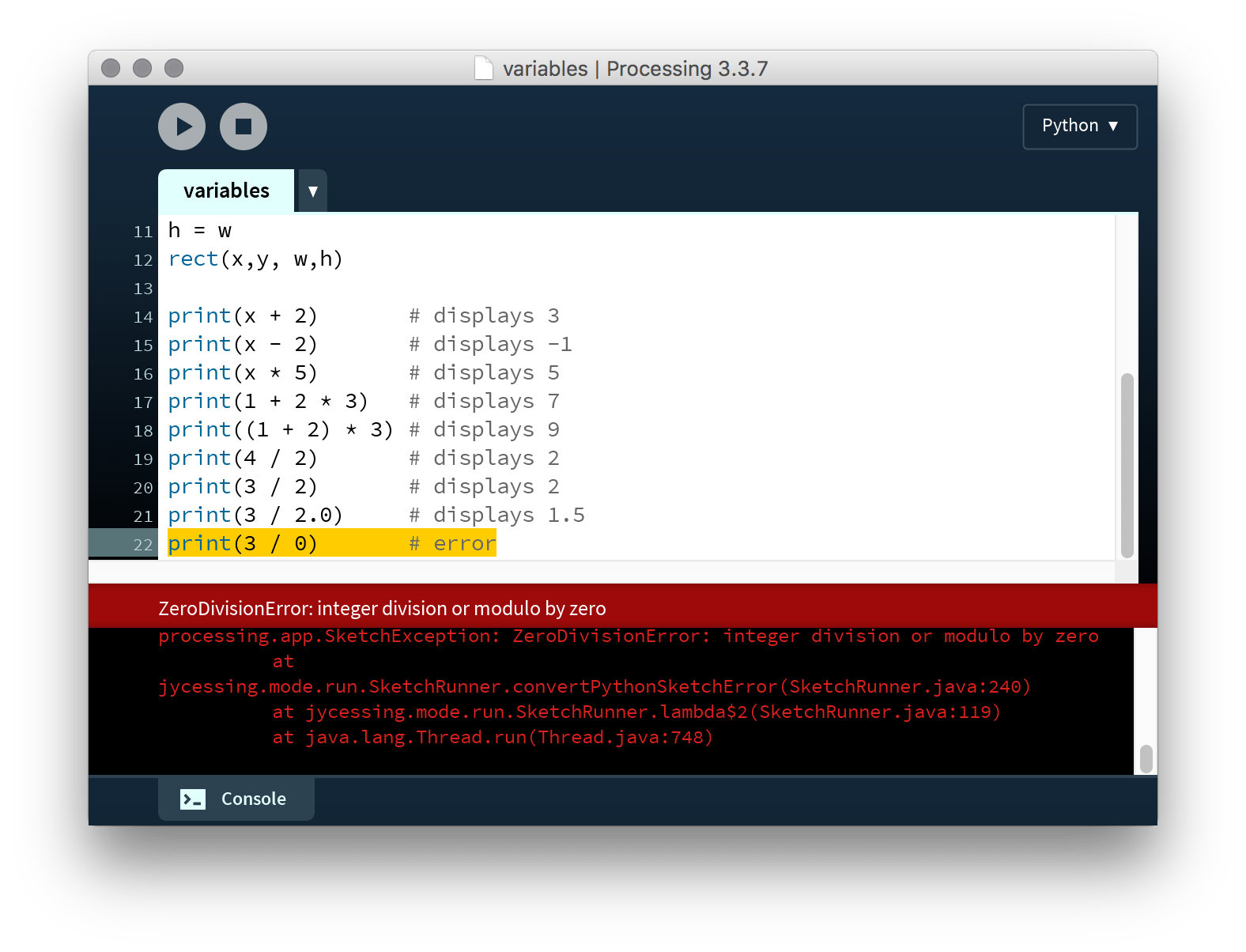
Modulo Operator
The modulo operator is written as a percentage sign (%
). It calculates the remainder of a division operation. Take five divided by two as an example:
- one could say the answer is
2.5
; - or, that the answer is
2
remainder1
, because two ‘goes into’ five twice with one left over.
The modulo operator performs the latter. Here’s some code contrasting a division and modulo operation:
print(5.0 / 2) # displays 2.5
print(5.0 % 2) # displays 1
It may not be evident why this is operator useful. However, many important algorithms – such as those used in cryptography – make use of it. For now, consider that modulo operations resulting in a 0
indicate that numbers divide exactly. Among other uses, this is handy for establishing whether a number is odd or even:
print(7 % 2) # displays 1, therefore 7 is odd
print(6 % 2) # displays 0, therefore 6 is even
You’ll be making use of modulo operators in future lessons.
Image Reveal Task
Time for another challenge!
The idea here’s to follow the instructions to reveal a symbol. Create a new sketch and save it as “symbol_reveal”. Add some code to get started:
size(600, 740)
background('#004477')
noFill()
stroke('#FFFFFF')
strokeWeight(3)
xco = 400
yco = 440
To begin revealing the symbol, follow instruction steps 1 to 6. To get you started, here’s the first instruction, along with the correct code:
- Draw a line beginning at an x-coordinate of half the display window
width
, and y-coordinate of a third of the windowheight
. The endpoint must have an x/y-coordinate equal toxco
&yco
.
– which will be coded as follows:
line(width/2,height/3, xco,yco)
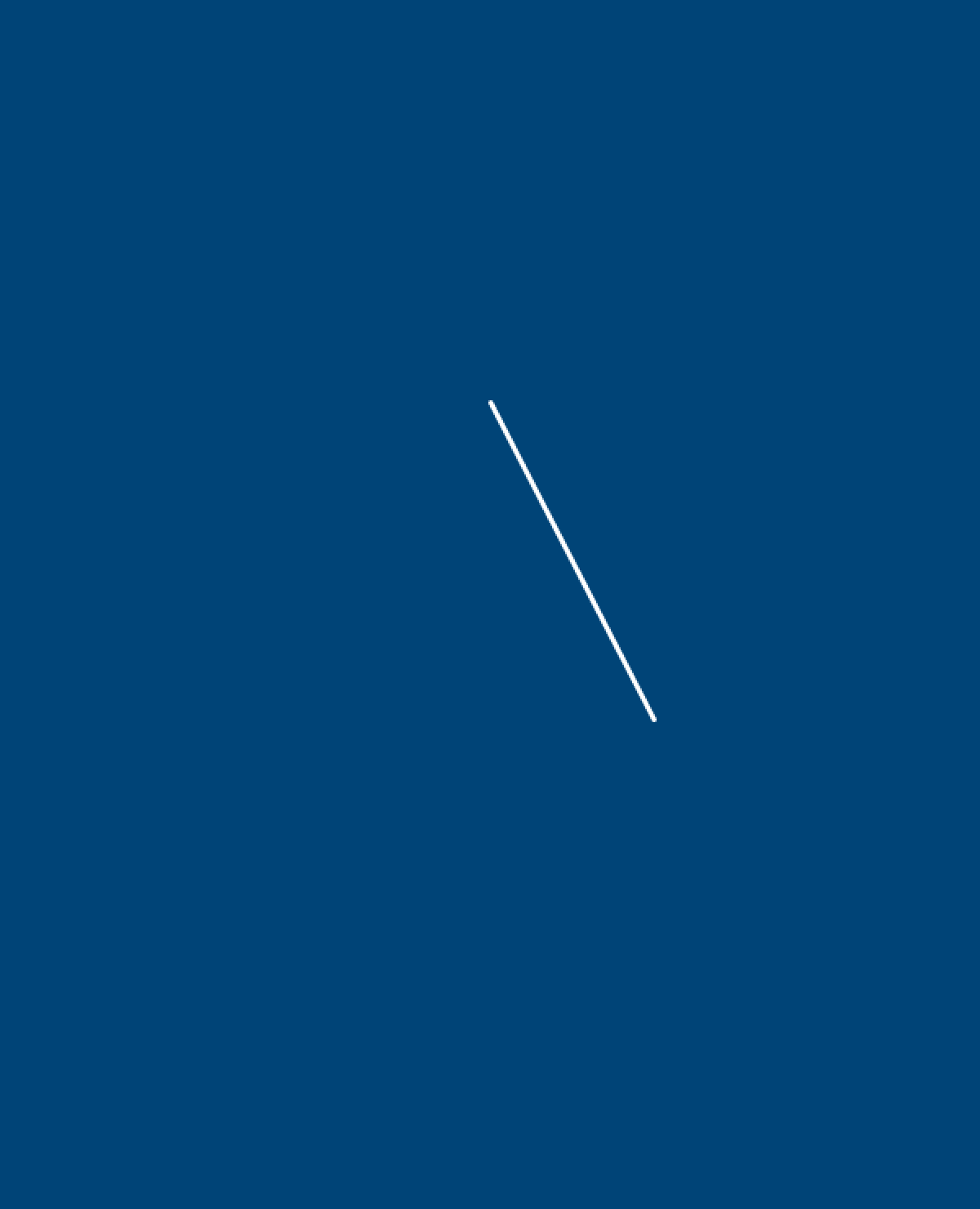
Now, carry-out the rest of the instructions:
-
Draw a centred ellipse with a width that's an eleventh of the display window
width
, and a height that's a fourteenth of the windowheight
. -
Draw a centred ellipse with a width that's a nineteenth of the display window
width
, and a height that's a twenty-second of the windowheight
. -
Draw a line beginning at an x/y-coordinate equal to
xco
&yco
respectively. The endpoint must have an x-coordinate of the display windowwidth
minusxco
, and a y-coordinate equal toyco
. -
Draw a line beginning at an x-coordinate of the display window
width
minusxco
, and y-coordinate equal toyco
. The endpoint must have an x-coordinate of half the display windowwidth
, and a y-coordinate of a third of the windowheight
. -
Draw a centred ellipse with a width that's a fifth of the display window
width
, and height that's a twelfth of the display windowheight
.
Clue: if this seems like a conspiracy, you may be on the right track.
1.6: Disk Space Analyser Task »
Complete list of Processing.py lessons
References
- http://py.processing.org/reference/