« 1.3: Drawing |
1.5: Arithmetic Operators »
Variables
Variables are placeholders for information – much like when you use letters in algebra to represent a value. In fact, Python variables look and behave similarly.
Begin a new sketch and then save it as “variables”. To keep things simple, we’ll print to the Console area. Add the following code:
size(600, 400)
background('#004477')
print(width)
print(height)
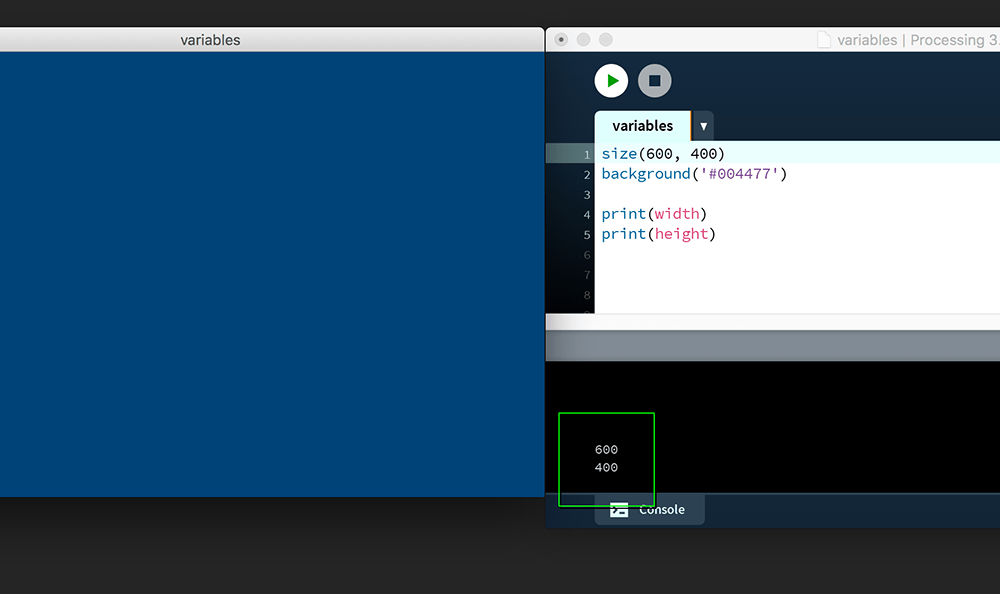
width
and height
are system variables that store the dimensions of the display window. However, you are not limited to system variables. When declaring your own variables, you assign a value using an =
sign (assignment operator). Try this out with a new variable named “x”:
...
x = 1
print(x) # displays 1 in the console
You may name your variables whatever you wish, provided the name: contains only alpha-numeric and underscore characters; does not begin with a number; and does not clash with a reserved Python keyword or variable (like width
). For example:
playerlives = 3 # correct
playerLives = 3 # correct
player_lives = 3 # correct
player lives = 3 # incorrect (contains a space)
player-lives = 3 # incorrect (contains a hyphen)
Whether you should name a variable using camelCase, underscores, or some other convention is a matter of style (and vociferous debate). However, it’s good to decide upon and stick to a naming convention, as you’ll make extensive use of variables in Processing. Add three more variables to your script, using them as arguments in a rect()
function:
...
x = 1
print(x) # displays 1 in the console
y = 30
w = 20
h = w
rect(x,y, w,h)
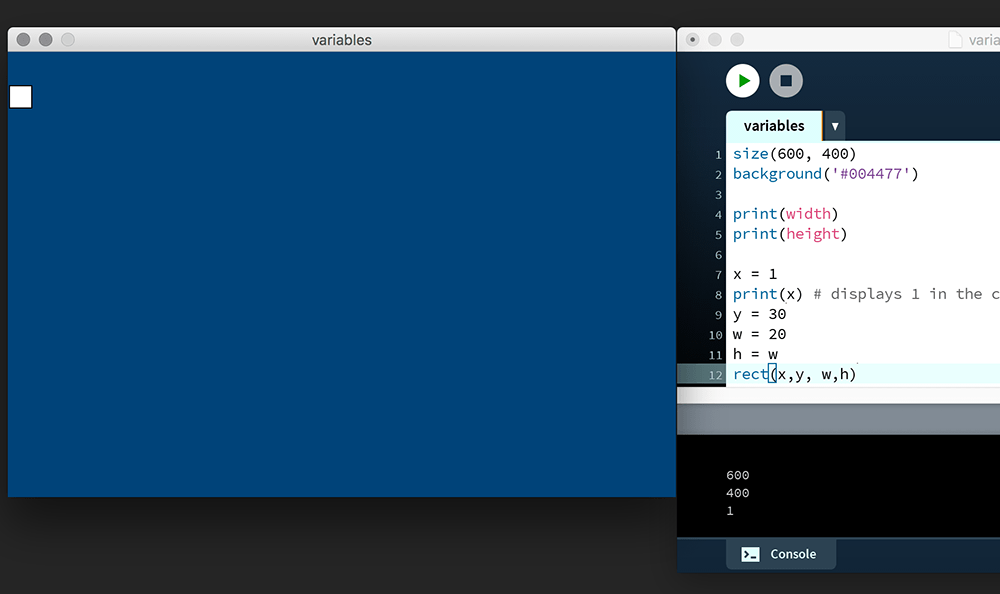
w
variable has been assigned to h
, resulting in a square.
1.5: Arithmetic Operators »
Complete list of Processing.py lessons