« 2.3: Strings |
2.5: Apple Logo Task »
Typography
With a good grasp of strings, you can move onto displaying text in the display window.
Typography refers to the arranging and styling of text (or, more correctly, type) to make it more legible, readable, and aesthetically appealing. Typographical treatment can truly make or break a design. Headings work best if they stand-out from the rest of your text; letter-spacing should be tighter than word-spacing; cursive fonts are not ideal for road signs.
Fonts
Early computer fonts were pixel-based, which required variant glyph sets for each font-size. However, modern fonts are vector-based, which is why you can scale text as large as you like without encountering any pixelation. Fonts must be loaded into Processing, but there’s a default sans-serif font should you not load any.
If you are unfamiliar with font classifications, serifs are the small lines attached to the tips of characters. By prefixing a term with “Sans”, one implies an absence of whatever follows it; hence a sans-serif fonts have no serifs.
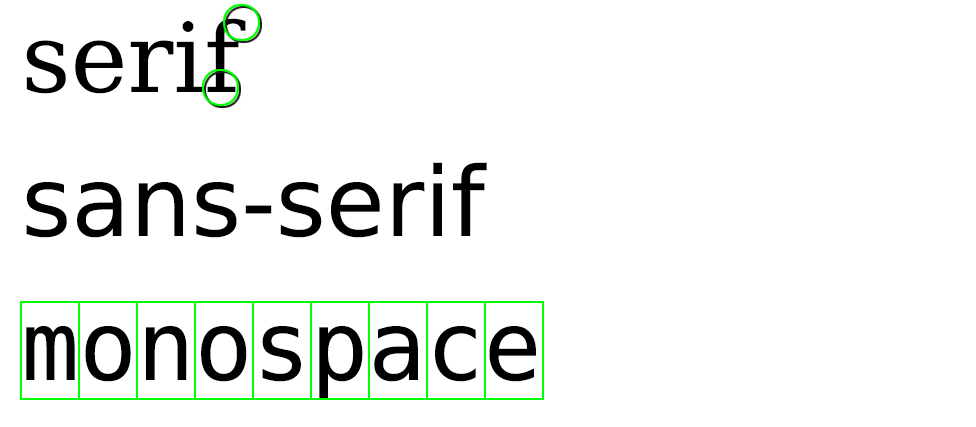
Monospaced fonts may also be serifed, but what defines them is how each character occupies the same amount of horizontal space. To make text more legible, (variable-width) fonts include metrics to specify how far a given character should sit from any neighbours. For example, having an “i” and “m” character occupy the same size container results in some awkward spacing issues – which many monospaced fonts attempt to resolve by adding enlarged serifs to the “i” and cramping the “m”:
monospaced
mmm
iii
variable-width
mmm
iii
That said, monospace fonts are more legible in certain situations – for instance, when it’s helpful to have characters line-up in columns:
monospaced
sam | jan | amy | tim
99 | 359 | 11 | 3
variable-width
sam | jan | amy | tim
99 | 359 | 11 | 3
This makes monospaced fonts preferable for source code, which is why the default font for the Processing editor (and every other code editor) is monospace.
Typography Sketch
Create a new sketch and save it as “typography”. Setup your sketch using the following code:
size(500, 500)
background('#004477')
fill('#FFFFFF')
stroke('#0099FF')
strokeWeight(3)
Now add a string variable (note: the line must not wrap):
razor = 'Never attribute to malice that which is adequately explained by stupidity.'
When you run the sketch, an empty blue display window appears. What follows below are descriptions for several typographic functions, along with some code to add to your working sketch. Feel free to experiment with the arguments to see how things respond.
text()
Draws text to the display window, the colour of which is determined by the active fill()
. The arguments represent the string value, x-coordinate, and y-coordinate respectively. Additional third and fourth argument can be added to specify a width and height for the text area.
Reference link: text()
text(razor, 0,50)
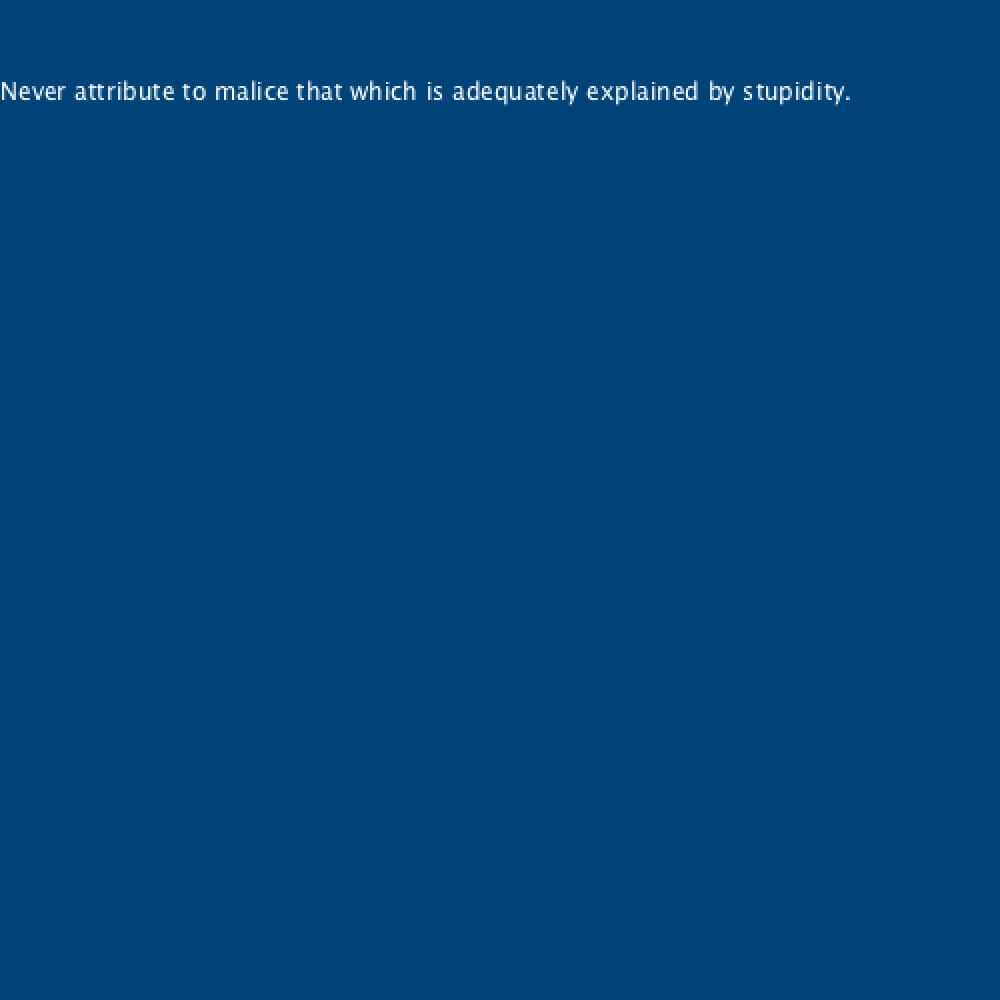
textSize()
Sets the font size (in pixels) to be used in all subsequent text()
functions.
Reference link: textSize()
textSize(20)
text(razor, 0,100)
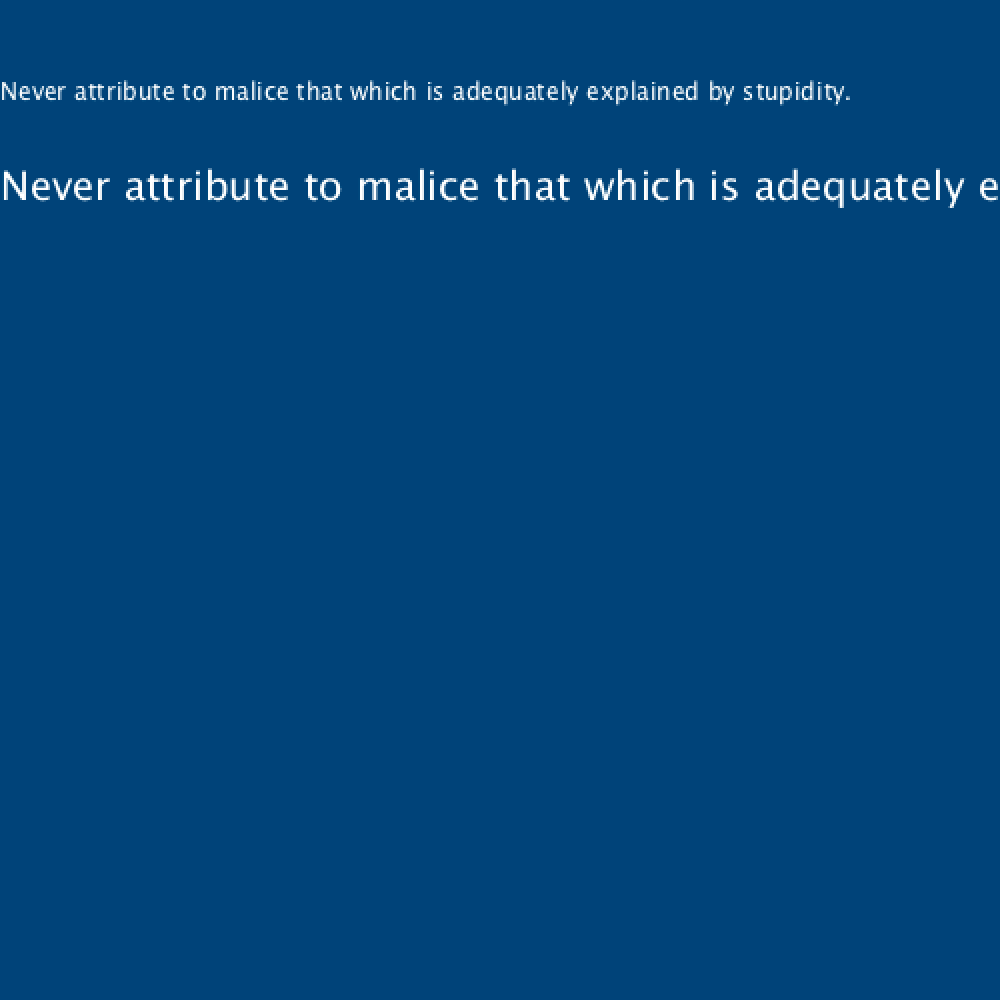
createFont()
Converts a font to the format used by Processing. The two arguments represent the font name and size, respectively. For a list of fonts available on your computer, use PFont.list()
. It’s probably a good idea to place the font files (TTF or OTF) in the sketch’s “data” directory, as not every computer is likely to have the font you’ve used installed. If you are loading fonts from the data directory, use the full file name (including the extension).
Reference link: createFont()
print( PFont.list() )
timesroman = createFont('Times-Roman', 20)
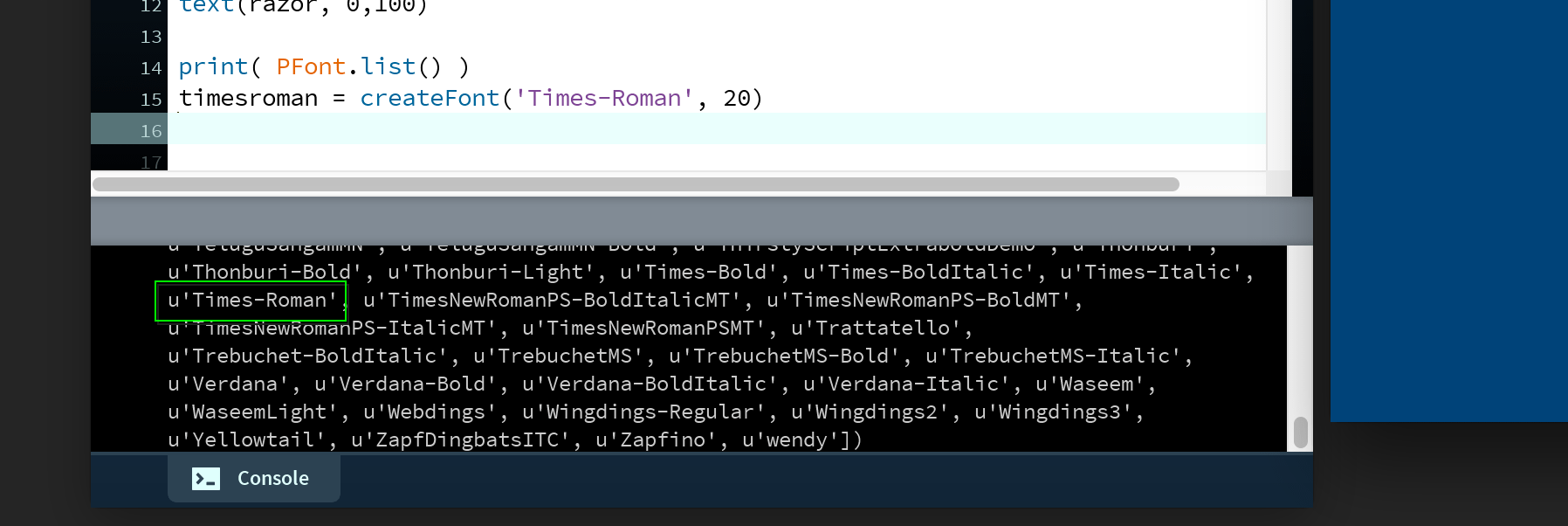
PFont.list()
textFont()
Sets the font for any subsequent text()
functions.
Reference link: textFont()
...
timesroman = createFont('Times-Roman', 20)
textFont(timesroman)
text(razor, 0,150)
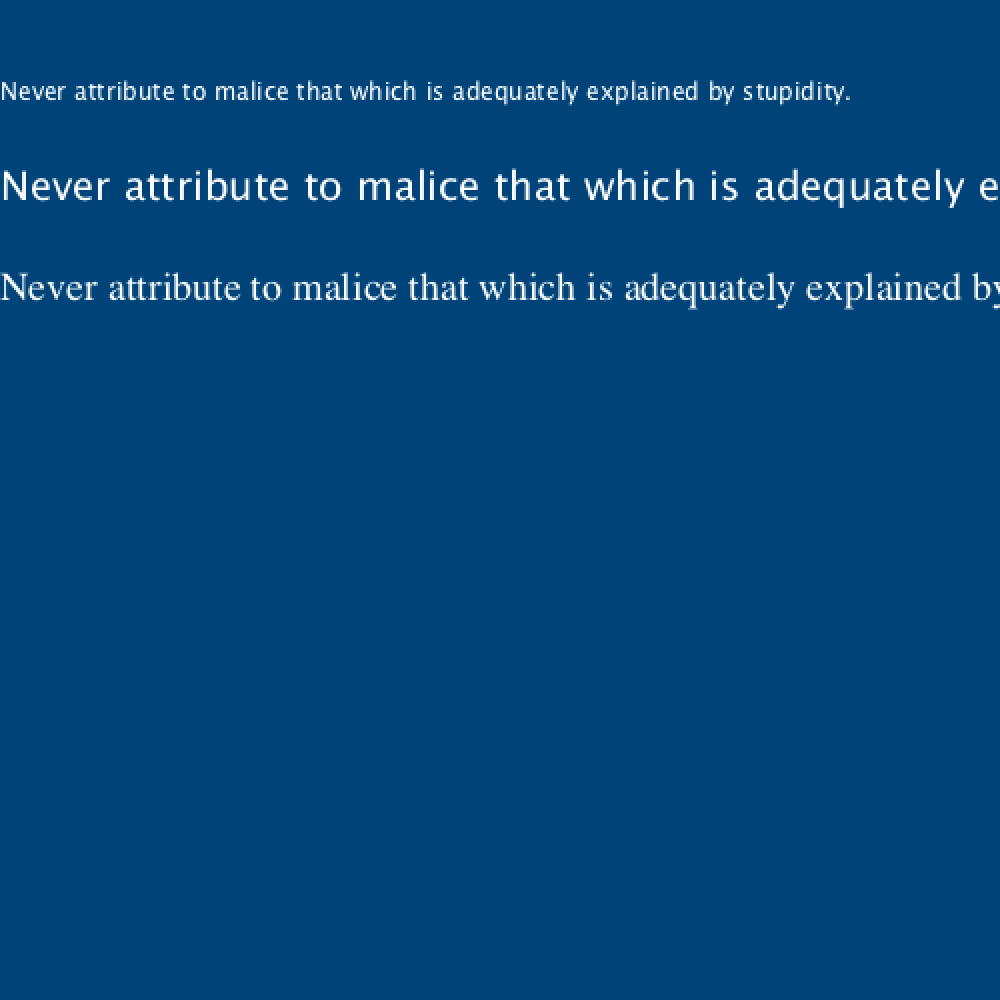
textLeading()
Sets the line-spacing (in pixels) for any subsequent text()
functions.
Reference link: textLeading()
textLeading(10)
text(razor, 0,200, 250,100)
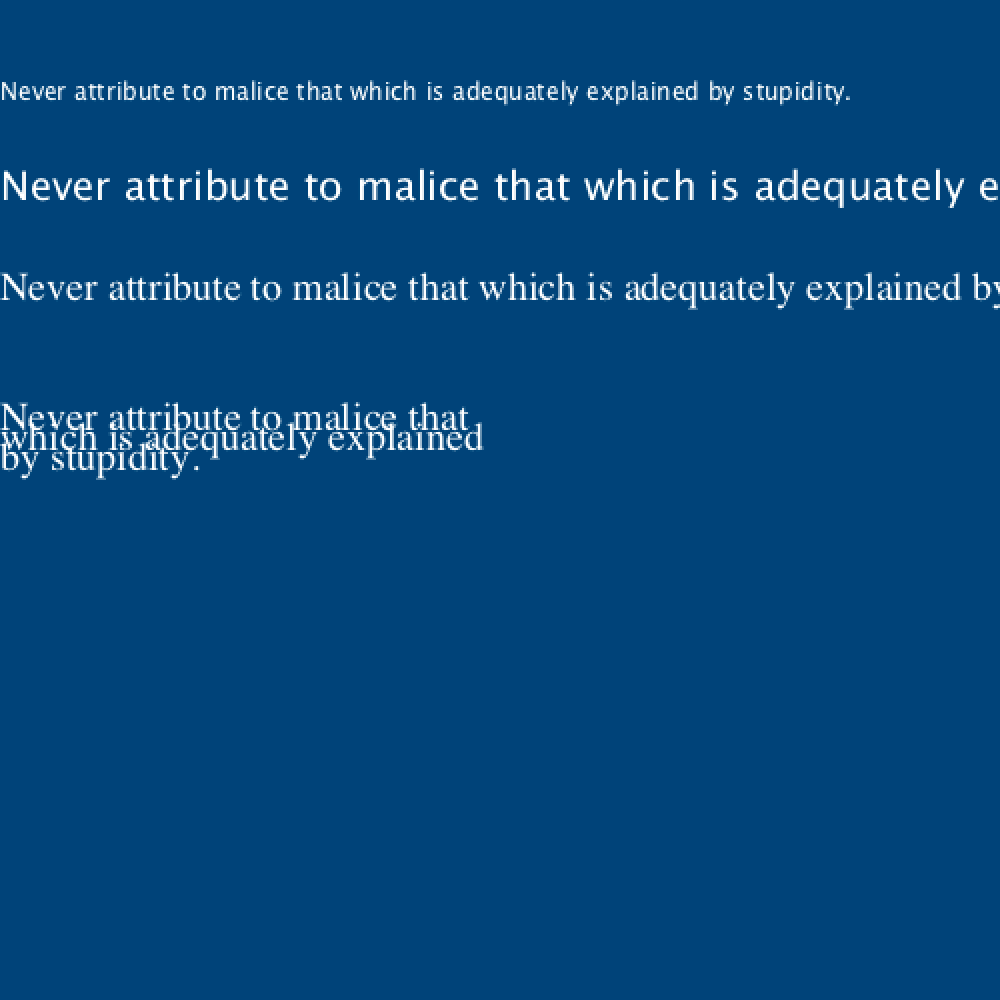
textAlign()
Sets the text-alignment for any subsequent text()
functions. Accepts the arguments LEFT
, CENTER
, or RIGHT
.
Reference link: textAlign()
textAlign(RIGHT)
text(razor, 0,250, 250,100)
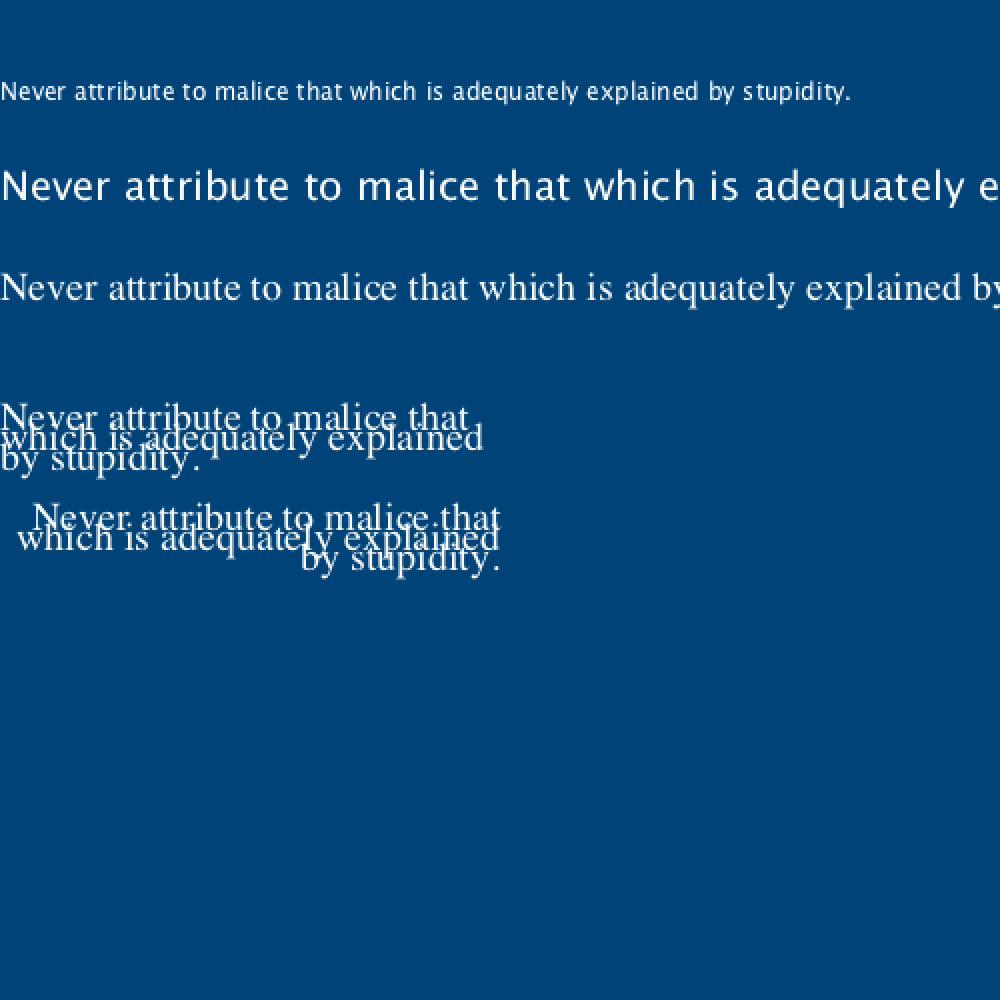
textWidth()
Calculates and returns the width of any string.
Reference link: textWidth()
textAlign(LEFT)
hanlons = '- Hanlon\'s'
razor = 'razor'
text(hanlons + ' ' + razor, 0,350)
line(
textWidth(hanlons), 0,
textWidth(hanlons), height
)
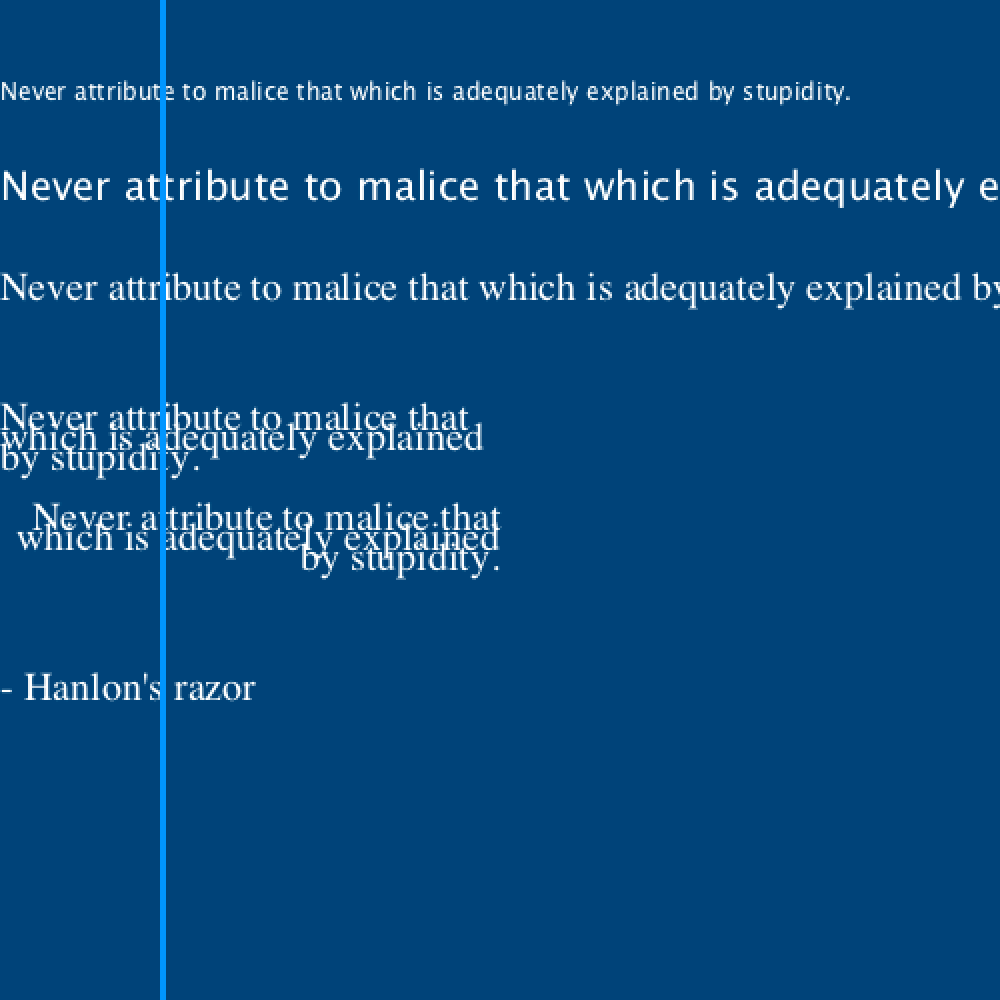
2.5: Apple Logo Task »
Complete list of Processing.py lessons